主要概念
► Data Binding
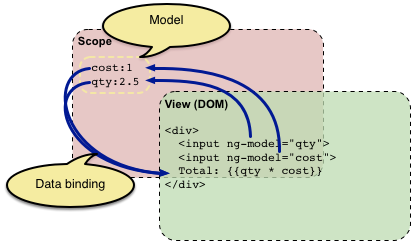
index.html
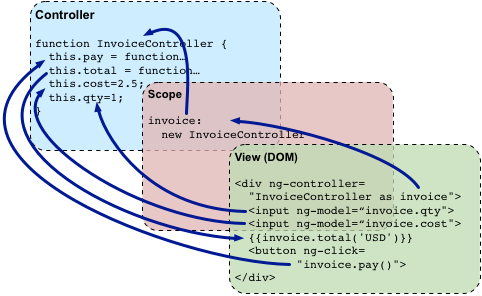
index.html
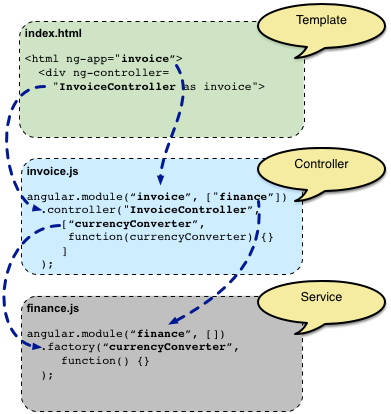
index.html 同上, 僅 ng-app設為 Invoice2, 並改引入 invoice2.js 如下
index.html 同上, 僅 ng-app設為 Invoice3, 並改引入 invoice3.js 如下
Concept | Description |
---|---|
Template | 放置 HTML 原始編碼, 提供後續的View呈現 |
Directives | 增加自訂的 HTML 元素或屬性 |
Model | 資料的存放與提供the data that is shown to the user and with which the user interacts |
Scope | 存放context 的模型, 讓 controllers, directives and expressions 等透過$scope來取寫 |
Expressions | 指讀寫 $scope 內的 variables 和 functions |
Compiler | 轉譯 template and instantiates directives and expressions |
Filter | 提供呈現給使用者的動態資料過濾功能 |
View | 呈現給使用者的資訊 (the DOM) |
Data Binding | model 和 view 雙向資料同步 |
Controller | the business logic behind views |
Dependency Injection | Creates and wires objects / functions |
Injector | dependency injection container |
Module | configures the Injector, angular.module , angular.Module , |
Service | reusable business logic independent of views |
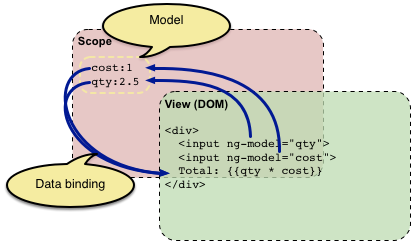
index.html
►使用控制器 UI logic: Controllers<!doctype html> <html><head><meta charset="UTF-8"> <script src="angular.js"></script> </head> <body ng-app=""> <div ng-init="qty=1;cost=2"> <b>Invoice:</b> <div> Quantity: <input type="number" ng-model="qty" required > </div> <div> Costs: <input type="number" ng-model="cost" required > </div> <div> <b>Total:</b> {{qty * cost | currency}} </div> </div> </body> </html>
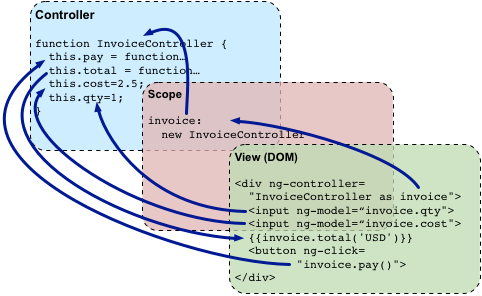
index.html
invoice1.js<html > <head> <script src="angular.js"></script> <script src="invoice1.js"></script> </head> <body ng-app="invoice1"><!-- 告知angular使用invoice1這個Module --> <div ng-controller="InvoiceController as invoice"> <b>Invoice:</b> <div> Quantity: <input type="number" ng-model="invoice.qty" required > </div> <div> Costs: <input type="number" ng-model="invoice.cost" required > <select ng-model="invoice.inCurr"> <option ng-repeat="c in invoice.currencies">{{c}}</option> </select> </div> <div> <b>Total:</b> <span ng-repeat="c in invoice.currencies"> {{invoice.total(c) | currency:c}} </span> <button class="btn" ng-click="invoice.pay()">Pay</button> </div> </div> </body> </html>
► 加入Service, View independent business logic: Servicesangular.module('invoice1', []) .controller('InvoiceController', function() { this.qty = 1; this.cost = 2; this.inCurr = 'EUR'; this.currencies = ['USD', 'EUR', 'CNY']; this.usdToForeignRates = { USD:1, EUR:0.74, CNY:6.09 }; this.total = function total(outCurr) { return this.convertCurrency(this.qty * this.cost, this.inCurr, outCurr); }; this.convertCurrency = function convertCurrency(amount, inCurr, outCurr) { return amount * this.usdToForeignRates[outCurr] / this.usdToForeignRates[inCurr]; }; this.pay = function pay() { window.alert("Thanks!"); }; });
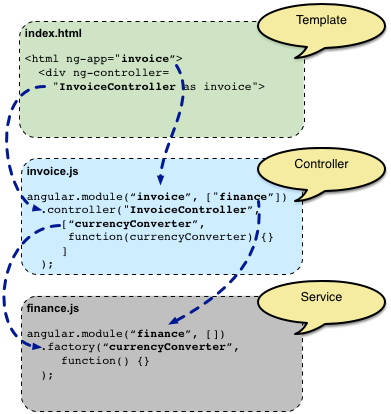
index.html 同上, 僅 ng-app設為 Invoice2, 並改引入 invoice2.js 如下
4. 遠端訪問 Accessing the backend//controller angular.module('invoice2', ['FinanceService']) .controller('InvoiceController', ['currencyConverter', function(currencyConverter) { this.qty = 1; this.cost = 2; this.inCurr = 'EUR'; this.currencies = currencyConverter.currencies; this.total = function total(outCurr) { return currencyConverter.convert(this.qty * this.cost, this.inCurr, outCurr); }; this.pay = function pay() { window.alert("Thanks!"); }; }]); // service angular.module('FinanceService', []) .factory('currencyConverter', function() { var currencyList = ['USD', 'EUR', 'CNY']; var usdToForeignRates = { USD: 1, EUR: 0.74, CNY: 6.09 }; var exchangeRate = function(amount, inCurr, outCurr) { return amount * usdToForeignRates[outCurr] / usdToForeignRates[inCurr]; }; //回傳可供外部使用的 屬性 或 函式 return { currencies: currencyList, convert: exchangeRate }; });
index.html 同上, 僅 ng-app設為 Invoice3, 並改引入 invoice3.js 如下
//controller angular.module('Invoice3', ['FinanceService']) .controller('InvoiceController', ['CurrencyConverter', function(currencyConverter) { this.qty = 1; this.cost = 2; this.inCurr = 'EUR'; this.currencies = currencyConverter.currencies; this.total = function total(outCurr) { return currencyConverter.convert(this.qty * this.cost, this.inCurr, outCurr); }; this.pay = function pay() { window.alert("Thanks!"); }; }]); // service angular.module('FinanceService', []) .factory('CurrencyConverter', ['$http', function($http) { var YAHOO_FINANCE_URL_PATTERN = 'http://query.yahooapis.com/v1/public/yql?q=select * from ' + 'yahoo.finance.xchange where pair in ("PAIRS")&format=json&' + 'env=store://datatables.org/alltableswithkeys&callback=JSON_CALLBACK'; var currencyList = ['USD', 'EUR', 'CNY', 'TWD']; var usdToForeignRates = {}; var exchangeRate = function(amount, inCurr, outCurr) { return amount * usdToForeignRates[outCurr] / usdToForeignRates[inCurr]; }; var refresh = function() { var url = YAHOO_FINANCE_URL_PATTERN.replace('PAIRS', 'USD' + currencyList.join('","USD')); return $http.jsonp(url).success(function(data) { var newUsdToForeignRates = {}; angular.forEach(data.query.results.rate, function(rate) { var currency = rate.id.substring(3, 6); newUsdToForeignRates[currency] = window.parseFloat(rate.Rate); }); usdToForeignRates = newUsdToForeignRates; }); }; refresh(); return { currencies: currencyList, convert: exchangeRate, refresh: refresh }; }]);